Here is a quick C# tip of counting up values of a Dictionary<TKey, TValue> where the values contains a List<T> using Linq. Lets say you have a Dictionary that contains Dictionary<string, List<Guid>> and you wish to find out how many items of Guid you have. You can easily do it by the Sum Linq aggregate […]
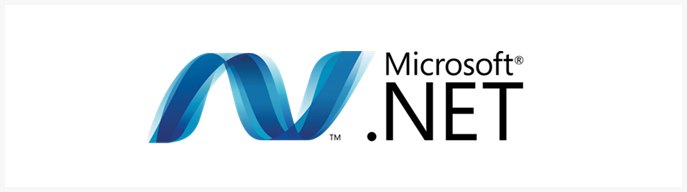
Here is a quick C# tip, when using string compare don’t use
1 2 3 |
var str = "Hello"; if(str.ToLower() === "hello") |
use the Equals and StringComparison.InvariantCultureIgnoreCase enum.
1 2 3 |
var str = "Hello"; if(str.Equals("hello", StringComparison.InvariantCultureIgnoreCase)) |
The thing you need to note is which rules are appropriate current culture, the invariant culture, ordinal or another culture entirely. One can create the compare from StringComparer.Create(culture, true)
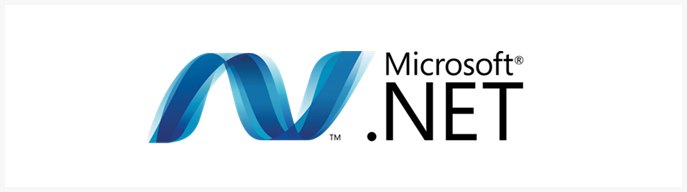
Here is another C# tip. Most of the time when you are trying to get a value from dictionary you would try to check if the key exist or not something like.
1 2 3 4 5 6 |
if(dictionary.ContainsKey(key)) { var value - dictionary[key]; //do some stuff } |
But one can use TryGetValue
1 2 3 4 |
if(dictionary.TryGetValue(key, out value)) { //do stuff with value } |
The benefit of using TryGetValue is that the dictionary is synchronized, there is no race condition.
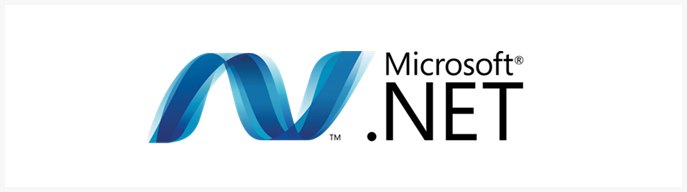
In C# there is a params keyword which allows a method parameter to take variable number of arguments. MSDN Some notes on param It must always be the last parameter in the function/method definition There can only be one params keyword in a function/method parameter signature Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
int Sum(int[] args) { int sum = 0; foreach (var item in args) { sum += item; } return sum; } //calling the above Sum method we would call it like int[] array = new int[] {1, 2, 3} int result = Sum(array); |
But by changing it to params we […]
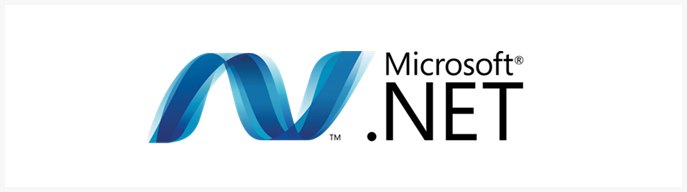
In C# there is the out parameter keyword which causes arguments to be passed by reference. It is like the ref keyword, except that ref requires that the variable be initialized before it is passed. Example:
1 2 3 4 5 6 7 8 9 10 |
void Init(out int x) { x = 47; } int x; Init(out x); Console.WriteLine(x); //x now is 47 |
The out parameter keyword provides a way for a method to output multiple values from a method without […]
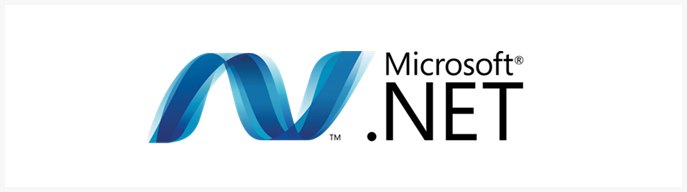
In C# when using the ref keyword it causes an argument to be passed by reference, not by value. In order to use a ref as a parameter, both the method definition and the calling method must explicitly use the ref keyword, and also the variable must be initialized before passing in. In the example […]
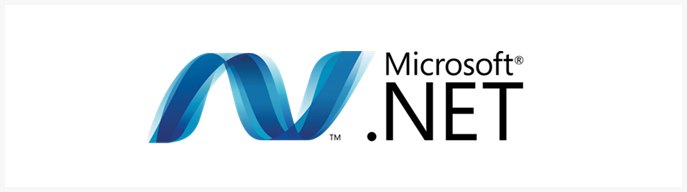
.NET provides in its System.IO namespace the Path class which performs operations on String instances that contain file or directory path information. These operations are performed in a cross-platform manner. Most of the time we see develeopers writing code like
1 |
string path = somePath + "\\" + filename; |
But by using Path.Combine we can provide a cross platform path
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
string somePath = @"C:\temp"; string filename = "dat.txt"; string path = Path.Combine(somePath,filename); //produce an output of C:\temp\dat.txt //linux: C:/temp/dat.txt string[] paths = {@"c:\My Music", "2013", "media", "banner"}; string fullPath = Path.Combine(paths); //output c:\My Music\2013\media\banner string path1 = @"C:\Temp"; string path2 = "My Music"; fullPath = Path.Combine(path1, path2); //output: C:\Temp\My Music fullPath = Path.Combine(string.Empty, path2); //output: My Music fullPath = Path.Combine(path1, string.Empty); //output: C:\Temp |
By using […]
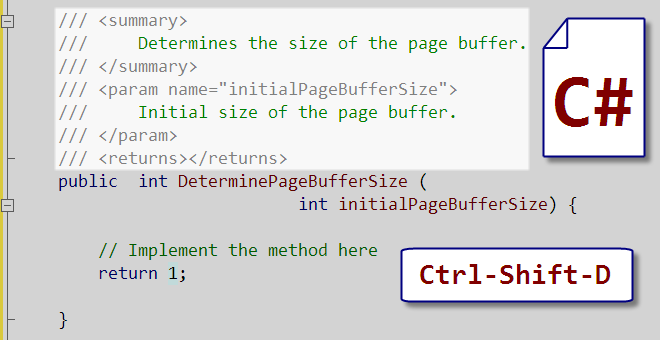
Recently have been going through some old code to review the comments in them from other developers and what I find out is developers tend to have really bad comments & documentation in their code Example:
1 2 3 4 5 6 7 8 |
public class Person { /// <summary> /// Constructor /// </summary> public Person() {} } |
From the above code it is obvious that it is the constructor but does the comment tell me […]
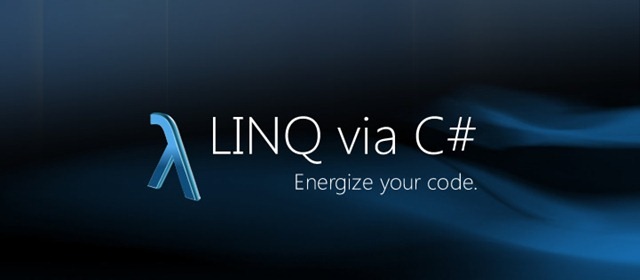
Here is a LINQ tip where you may wish to order a collection with an existing ordering of another collection. Example:
1 2 3 4 5 6 7 8 9 |
int[] displaySeq = new int[] { 1, 8, 5, 7, 13 }; //the ordering we want at the end //collection that we will be sorting on List<Person> people = new List<Person>(); people.Add(new Person { DisplaySeq = 5}); people.Add(new Person { DisplaySeq = 13}); people.Add(new Person { DisplaySeq = 7}); people.Add(new Person { DisplaySeq = 1}); people.Add(new Person { DisplaySeq = 8}); |
Currently as it stands our data is stored in the order of { 5, 13, 7, 1, 8 } and we wish to order them in terms of { 1, 8, 5, 7, […]