Here is a quick C# tip of counting up values of a Dictionary<TKey, TValue> where the values contains a List<T> using Linq. Lets say you have a Dictionary that contains Dictionary<string, List<Guid>> and you wish to find out how many items of Guid you have. You can easily do it by the Sum Linq aggregate […]
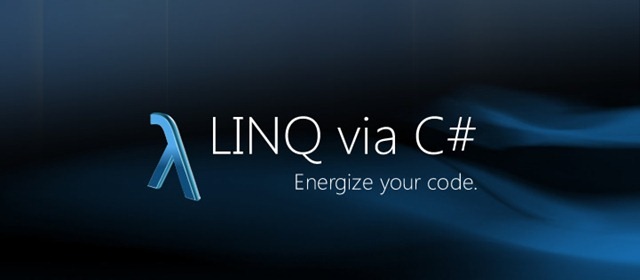
Here is a LINQ tip where you may wish to order a collection with an existing ordering of another collection. Example:
1 2 3 4 5 6 7 8 9 |
int[] displaySeq = new int[] { 1, 8, 5, 7, 13 }; //the ordering we want at the end //collection that we will be sorting on List<Person> people = new List<Person>(); people.Add(new Person { DisplaySeq = 5}); people.Add(new Person { DisplaySeq = 13}); people.Add(new Person { DisplaySeq = 7}); people.Add(new Person { DisplaySeq = 1}); people.Add(new Person { DisplaySeq = 8}); |
Currently as it stands our data is stored in the order of { 5, 13, 7, 1, 8 } and we wish to order them in terms of { 1, 8, 5, 7, […]
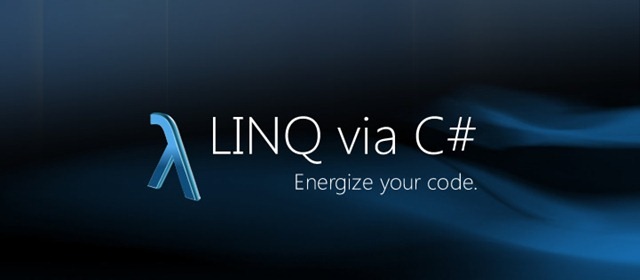
Was going through some legacy code recently and found a big for loop that had multiple switch statement inside. So being that I hate something that is fairly large, every method should be small and concise, I went along to refactor it to SRP. This code is generic so you might see something like this […]
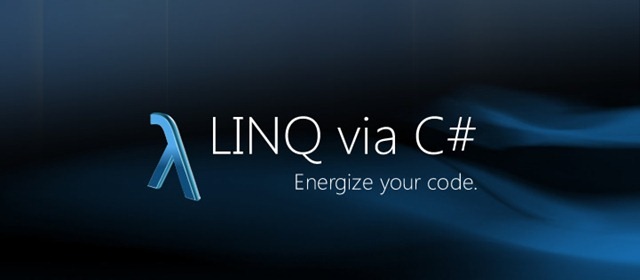
Here is some poorly written code that I had to review today and modify (i.e refactor). I have removed the domain of the code and renamed the variables, so that it remains anonymous, but the just of the code is still intact.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
var itemCount = 0; if (users.ShoppingCart != null && user.ShoppingCart > 0) { foreach (var cart in user.ShoppingCart) { if (cart.Items != null && cart.Items.Count > 0) { foreach (var item in cart.Items) { itemCount++; } } } } |
Lets go through the inner loop of the code first. First the […]
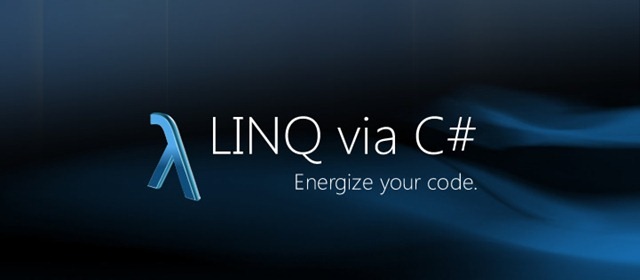
So sometimes you will get these strange errors or exceptions when using Linq Sequence contains no matching element For example if you use
1 |
var result = myEnumerableCollection.First(x => x.Name == person.Name); |
This will throw InvalidOperationException with Sequence contains no matching element. But if you use
1 2 3 |
var result = myEnumerableCollection.FirstOrDefault(x => x.Name == person.Name); if(result == null) //did not find in the collection something went wrong or ..... |
Which will return you a null if its not found then you can check if the […]