In interviews there is always the Singleton question, I personally refuse to answer it on interview. The reason is very simple I don’t code singletons anymore. I use my IoC Container to provide me with a singleton. Sorry if you dont know what an IoC (Inversion of Control Container) is, then you are still in […]
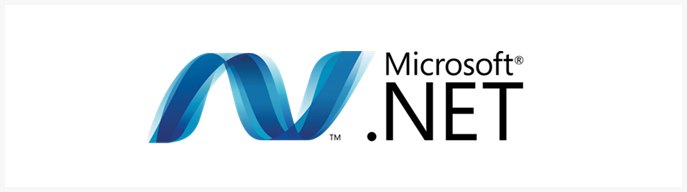
C# has the as keyword, it is mainly used to cast object of one type to another, and if it fails it should return a null rather than crashing the program or throwing exception. From MSDN documentation it states: You can use the as operator to perform certain types of conversions between compatible reference types […]
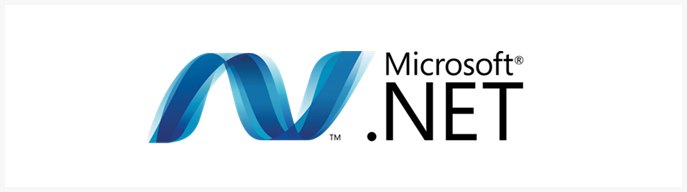
So C# what is the yield keyword? The yield keyword in C# is basically an iterator, its a form of syntactic sugar added from C# 2.0 to create IEnumerable and IEnumerator objects, thus returning one element at a time. From MSDN documentation it states. When you use the yield keyword in a statement, you indicate […]
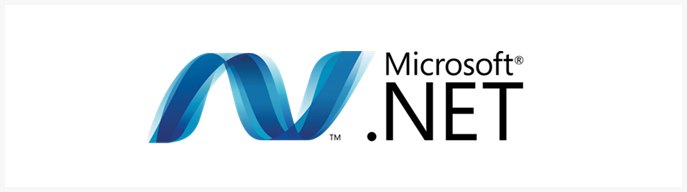
C# readonly what is that for? The readonly keyword in C# is used as a modifier. A field can be declared with readonly modifier, and its assignment can only be in the Constructor or when the variable is declared. An example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
public readonly int MinAge = 10; //this is valid public class Person { public readonly int _age; public Person(int age) { _age = age; //this is also valid } public void SetAge(int age) { _age = age; //this is invalid, one cannot set it here would give compile error } public int Age { get { return _age; } //this is also valid no setter just getter } } |
The main thing to understand about readonly modifiers is that they are initialized […]
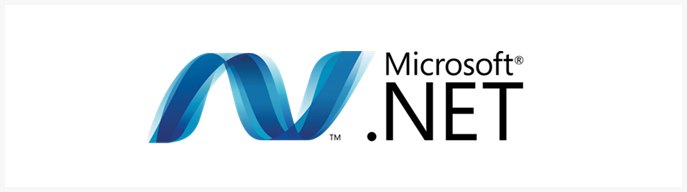
From the C# documentation it states that the @ symbol is an Identifier. 2.4.2 Identifiers The prefix “@” enables the use of keywords as identifiers, which is useful when interfacing with other programming languages. The character @ is not actually part of the identifier, so the identifier might be seen in other languages as a […]
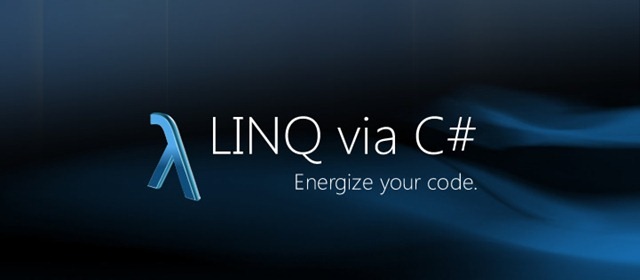
So sometimes you will get these strange errors or exceptions when using Linq Sequence contains no matching element For example if you use
1 |
var result = myEnumerableCollection.First(x => x.Name == person.Name); |
This will throw InvalidOperationException with Sequence contains no matching element. But if you use
1 2 3 |
var result = myEnumerableCollection.FirstOrDefault(x => x.Name == person.Name); if(result == null) //did not find in the collection something went wrong or ..... |
Which will return you a null if its not found then you can check if the […]