Was going through some legacy code recently and found a big for loop that had multiple switch statement inside. So being that I hate something that is fairly large, every method should be small and concise, I went along to refactor it to SRP. This code is generic so you might see something like this […]
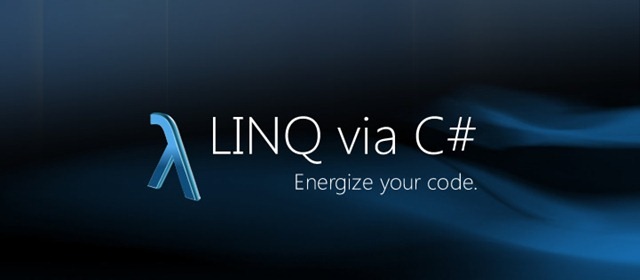
Here is some poorly written code that I had to review today and modify (i.e refactor). I have removed the domain of the code and renamed the variables, so that it remains anonymous, but the just of the code is still intact.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
var itemCount = 0; if (users.ShoppingCart != null && user.ShoppingCart > 0) { foreach (var cart in user.ShoppingCart) { if (cart.Items != null && cart.Items.Count > 0) { foreach (var item in cart.Items) { itemCount++; } } } } |
Lets go through the inner loop of the code first. First the […]
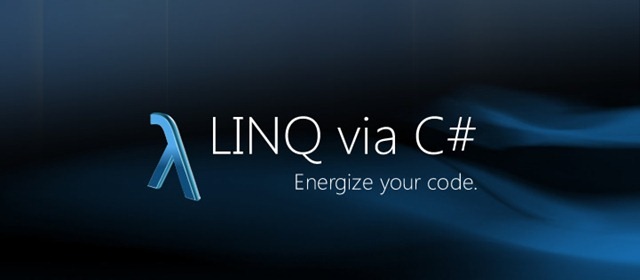
Operator ‘==’ cannot be applied to operands of type ‘System.Collections.Generic.KeyValuePair‘ and ‘‘
You are here beacause you probably got a Linq error, specifically Operator ‘==’ cannot be applied to operands of type ‘System.Collections.Generic.KeyValuePair‘ and ‘‘ So if you wish to do something like this to KeyValuePair using Linq
1 2 3 4 5 6 |
var item = myDictionary.FirstOrDefault(x => x.Key == 123); if(item != null) { //blah blah } |
You will get this error Operator ‘!=’ cannot be applied to operands of type ‘System.Collections.Generic.KeyValuePair‘ and ‘‘ Since […]