I wanted to start a series of blog post, maybe later include videos also on Azure OpenAI specifically for .NET Developers. Developers who use C# as their main languge of choice. The reason is I see lots of Python examples out there and felt a market was left out for most of the examples on […]
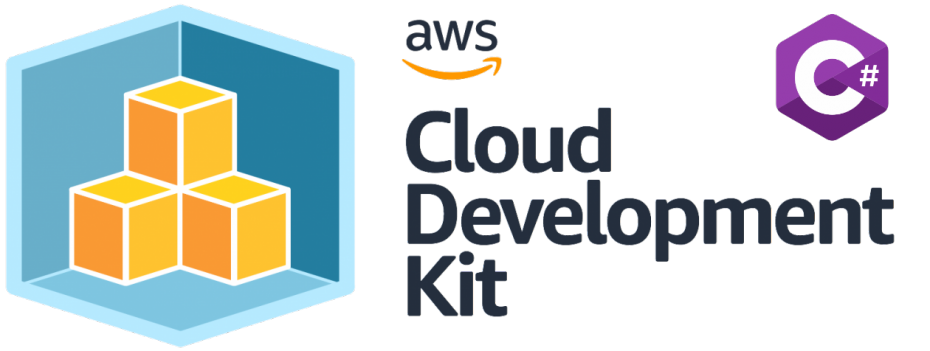
Now that we have learned how to create a VPC with C# using AWS CDK, let’s talk about how do we create RDS Database Instance with C# using AWS CDK. What is Amazon RDS? Amazon Relational Database Service (Amazon RDS) in simple terms is easy to set up, operate, and scale relational database. It provides […]
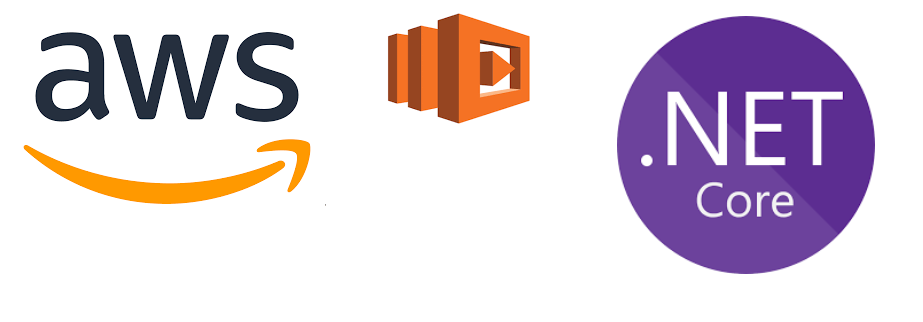
If you are using AWS S3 C# TransferUtilityUploadRequest and when you try to upload objects onto S3 you can potentially get Access Denied. The reason could your IAM Role is not defined to have access or your bucket name is incorrect. What do you mean bucket name is incorrect? Basically it boils down to that […]
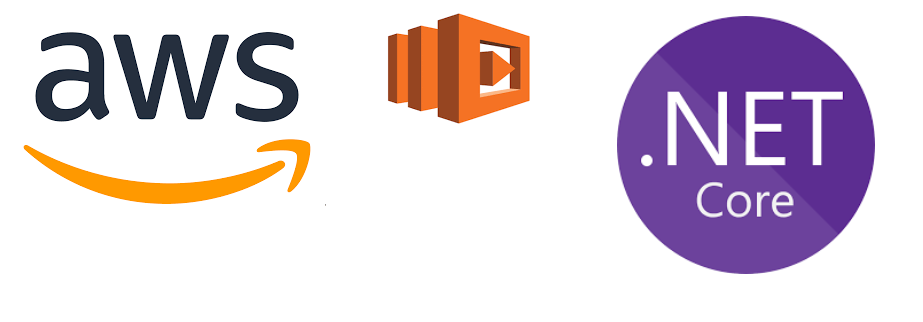
If you are working on AWS Lambda Dotnet with C# and find out that you are getting something like an error like below. The main reason is that you most likely created the lambda on the console first and then tried to upload the code using dotnet cli e.g dotnet lambda deploy-function “functionName”. In order […]
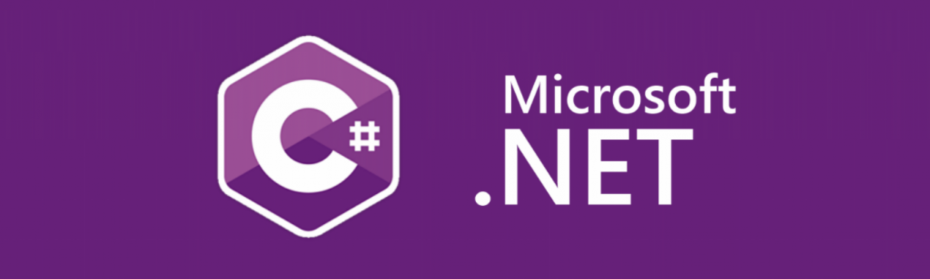
In C#7 there is an enhancement in the main method Async Main in C# that will allow you to await in your main method. Let me show you an example of how it was in C#6 and then how it has changed in C#7. You will remember this most likely
1 2 3 4 |
static int Main() { return SomeAsyncCall().GetAwaiter().GetResult(); } |
One will need to […]
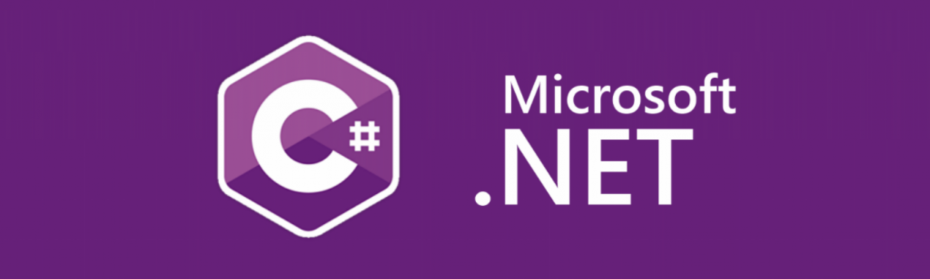
In C#7 there is an improvement on using out parameter. Some of you may remember writing code like below.
1 2 3 4 5 6 7 |
string hourString = "5"; int hour; if (int.TryParse(hourString, out hour)) { Console.WriteLine($"The hour is {hour}"); } |
The improvement that C#7 brings is you can now declare out variables in the argument list of a method call, rather than writing a separate declaration statement like below:
1 2 3 4 5 6 |
string hourString = "5"; if (int.TryParse(hourString, out int hour)) { Console.WriteLine($"The hour is {hour}"); } |
And it doesn’t end there […]