C# Func vs. Action? What is the difference between a Func vs an Action in C#? The main difference is whether one wants the delegate to return a value or not. By using Func it will expect the delegate to return a value and Action for no value (void) 1. Func for methods that return […]
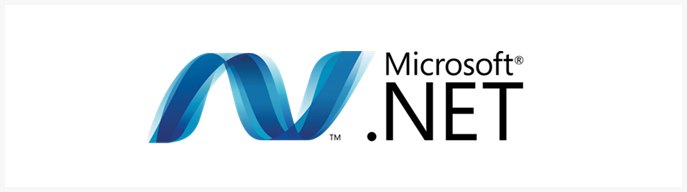
Lots of developers confuse the var keyword in C# with JavaScript. The var keyword was introduced in C# 3.0, and it is basically implicitly defined but is statically defined, var are resolved at compile time not at run-time. The confusion is there also a var keyword in JavaScript, but in JavaScript var is dynamically defined. […]
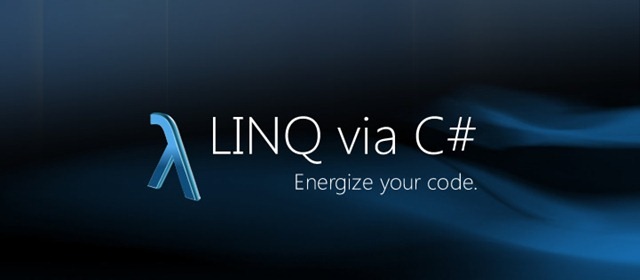
Was going through some legacy code recently and found a big for loop that had multiple switch statement inside. So being that I hate something that is fairly large, every method should be small and concise, I went along to refactor it to SRP. This code is generic so you might see something like this […]
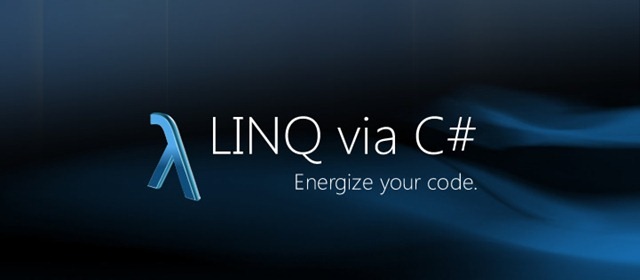
Here is some poorly written code that I had to review today and modify (i.e refactor). I have removed the domain of the code and renamed the variables, so that it remains anonymous, but the just of the code is still intact.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
var itemCount = 0; if (users.ShoppingCart != null && user.ShoppingCart > 0) { foreach (var cart in user.ShoppingCart) { if (cart.Items != null && cart.Items.Count > 0) { foreach (var item in cart.Items) { itemCount++; } } } } |
Lets go through the inner loop of the code first. First the […]
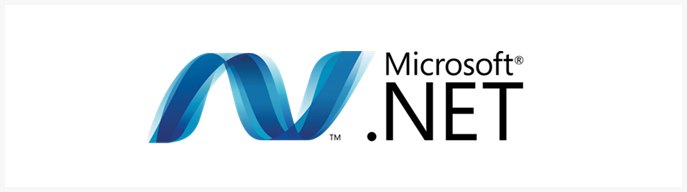
Here is a quick way to detect if a text is in Canadian Aboriginal Syllabic in C#. It is just an extension method that would detect if your string is in that range by using regex.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
public static bool IsInuktitut(this string input) { if (string.IsNullOrEmpty(input)) { return false; } //http://msdn.microsoft.com/en-us/library/20bw873z.aspx //look at section Supported Named Blocks const string pattern = @"\p{IsUnifiedCanadianAboriginalSyllabics}"; Match match = Regex.Match(input, pattern); return match.Success; } |
Enjoy.
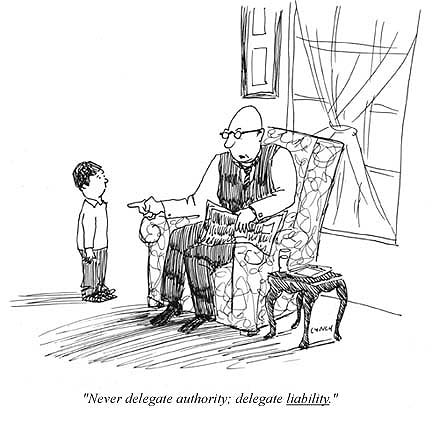
Here is a nice little code I did recently, where the Command Pattern is used with a notification observer like pattern. First of the Command Pattern, a simple interface for task with an execute method
1 2 3 4 5 6 7 |
public interface ITask { /// <summary> /// Execute the task /// </summary> void Execute(); } |
Then the notification interface, with 2 methods, one when it started and one when its complete and the event […]
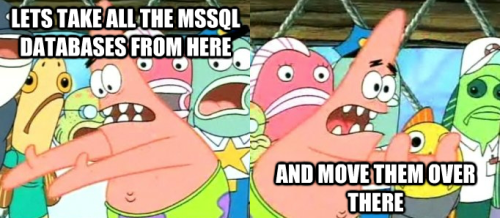
So was hacking a new installer and wanted to display the data link properties dialog, for building a connection string. Here is what I have found out by playing with it. One has to add the reference to ADODB.DLL (from .NET reference) and Microsoft OLE DB Service Component 1.0 Type Library from the COM tab […]