In interviews there is always the Singleton question, I personally refuse to answer it on interview. The reason is very simple I don’t code singletons anymore. I use my IoC Container to provide me with a singleton. Sorry if you dont know what an IoC (Inversion of Control Container) is, then you are still in […]
Tag: Design Pattern
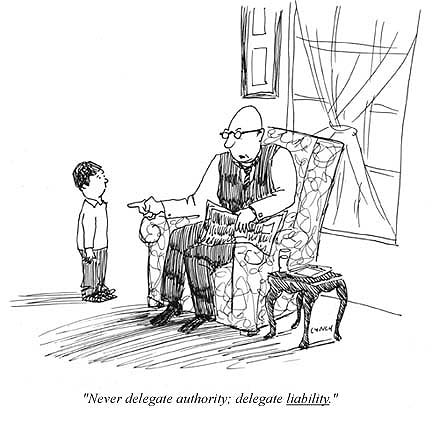
Here is a nice little code I did recently, where the Command Pattern is used with a notification observer like pattern. First of the Command Pattern, a simple interface for task with an execute method
1 2 3 4 5 6 7 |
public interface ITask { /// <summary> /// Execute the task /// </summary> void Execute(); } |
Then the notification interface, with 2 methods, one when it started and one when its complete and the event […]
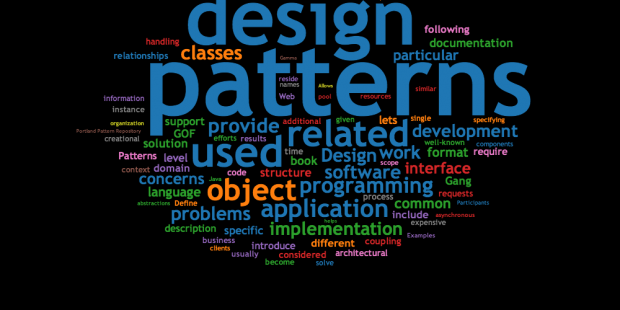
Null Pattern Last time I blogged about “Learn the Null Pattern“, but I forgot to mention one thing about the Null Pattern. That is you have to be mindful of it. For example
1 2 3 4 5 6 |
foreach(var id in IDList) { var employee = db.GetEmployee(id); salary = db.CalculateSalary(id); employee.Pay(salary); } |
The code looks okay but what if the GetEmployee method actually returns a NullEmployee? Well that would still be okay, since […]