Visual Studio Code with Python Flask
I wanted to continue on my python exploration and show how to get started with Visual Studio Code with Python Flask.
What is flask you may ask
Flask is a microframework for Python based on Werkzeug, Jinja 2 and good intentions. And before you ask: It’s BSD licensed!
Basically you can think of Flask somewhat like NancyFx or Sinatra web framework.
First thing first, in python we should set up our virtualenv such that we have a dev environment and don’t pollute our global space with our packages. You should already have pip install with your python if you are using the Python 3.x series.
Open up your powershell or command prompt and type
1 2 3 4 5 6 |
PS C:\Users\flask-sample> pip install virtualenv Collecting virtualenv Downloading virtualenv-15.1.0-py2.py3-none-any.whl (1.8MB) 100% |################################| 1.8MB 325kB/s Installing collected packages: virtualenv Successfully installed virtualenv-15.1.0 |
Once you have virtualenv we will need virtualenvwrapper-win since we are running under windows.
1 2 3 4 5 6 7 8 |
PS C:\Users\flask-sample> pip install virtualenvwrapper-win Collecting virtualenvwrapper-win Downloading virtualenvwrapper-win-1.2.1.zip Requirement already satisfied: virtualenv in c:\users\taswar\appdata\local\programs\python\python35-32\lib\site-packages (from virtualenvwrapper-win) Installing collected packages: virtualenvwrapper-win Running setup.py install for virtualenvwrapper-win ... done Successfully installed virtualenvwrapper-win-1.2.1 |
Now we need to create the virtualenv that we will be using.
1 |
PS C:\Users\flask-sample> mkvirtualenv FlaskProject |
This will create your virtualenv inside your home directory for mine it is under
1 |
PS C:\Users\taswar\Envs\FlaskProject\ |
In order to activate the virtualenv we need to use the activate.ps1 script but you must make sure we have the correct execution-policy in your powershell but before we do that lets launch our Vscode
1 2 3 4 |
PS C:\Users\taswar\Documents\GitHub\>mkdir flaskExample PS C:\Users\taswar\Documents\GitHub\>cd flaskExample PS C:\Users\taswar\Documents\GitHub\flaskExample>code . #Once in your vscode launch your command powershell prompt Ctrl+Shift+` |
Lets now set up our directory to use the virtualenv, remember the Set-ExecutionPolicy for powershell and use the terminal inside your vscode with Ctrl+Shift+` (right tick)
1 2 3 4 5 6 7 8 9 10 11 12 13 |
PS C:\Users\taswar\Envs\FlaskProject\Scripts\Activate.ps1 #one can set the project also (flaskProject) PS C:\Users\taswar\Documents\GitHub\flaskExample> setprojectdir . "C:\Users\taswar\Documents\GitHub\flaskExample" is now the project directory for virtualenv "C:\Users\taswar\Envs\flaskProject" "C:\Users\taswar\Documents\GitHub\flaskExample" added to C:\Users\taswar\Envs\flaskProject\Lib\site-packages\virtualenv_path_extensions.pth #once active you will see your prompt change (flaskProject) PS C:\Users\taswar\Documents\GitHub\flaskExample> |
Lets now install flask
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
#now lets install flask (flaskProject) PS C:\Users\taswar\Documents\GitHub\flaskExample>pip install flask Downloading Werkzeug-0.12.1-py2.py3-none-any.whl (312kB) 100% |################################| 317kB 585kB/s Collecting Jinja2>=2.4 (from flask) Downloading Jinja2-2.9.6-py2.py3-none-any.whl (340kB) 100% |################################| 348kB 926kB/s Collecting MarkupSafe>=0.23 (from Jinja2>=2.4->flask) Downloading MarkupSafe-1.0.tar.gz Building wheels for collected packages: itsdangerous, MarkupSafe Running setup.py bdist_wheel for itsdangerous ... done Stored in directory: C:\Users\taswar\AppData\Local\pip\Cache\wheels\fc\a\66\24d655233c757e178d45dea2de22a04c6d92766abfb741129a Running setup.py bdist_wheel for MarkupSafe ... done Stored in directory: C:\Users\taswar\AppData\Local\pip\Cache\wheels\88\a7\30\e39a54a87bcbe25308fa3ca64e8ddc75d9b3e5afa21ee32d57 Successfully built itsdangerous MarkupSafe Installing collected packages: click, itsdangerous, Werkzeug, MarkupSafe, Jinja2, flask Successfully installed Jinja2-2.9.6 MarkupSafe-1.0 Werkzeug-0.12.1 click-6.7 flask-0.12.1 itsdangerous-0.24 |
Lets now create a hello.py example of flask application, remember spaces are important for python
1 2 3 4 5 6 |
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' |
Go back to your powershell prompt and enter. (NOTE: you may need to restart vscode for this to work)
1 2 3 4 |
setx FLASK_APP = hello.py (flaskProject) PS C:\Users\taswar\Documents\GitHub\flaskExample> python -m flask run * Serving Flask app "hello" * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit) |
When everything is up and running you should be able to go to Chrome or your favourite browser and view port 5000 and see Hello world there.
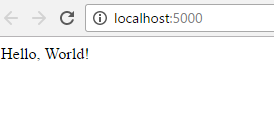
VsCode-Python-Flask-1
Hope this helps you in setting up vscode with development of Python Flask.
To learn about running VSCode with python read my previous post on Getting Started with Visual Studio Code with Python.