I needed to increase my disk size of my Windows 2016 server on AWS EC2. I though I will share this just in case it can help you or myself in the future on How to increase the disk size of a Windows EC2 machine?. First thing first you need to increase the volume space that you have, easiest way is to use the aws console.
Below I have logged in and am on the windows ec2 machine and have clicked on storage tab to find the volume I wish to increase.
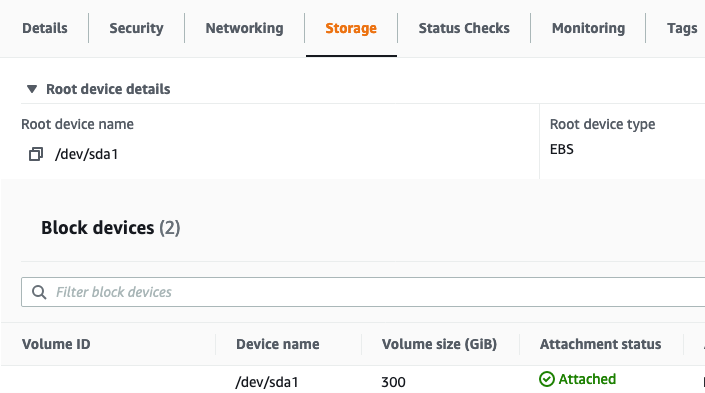
EC2-Stoage-Tab
Click on the storage volume link and it will take you to the storage section on the console. Right click and choose Modify Volume.
A window will show up like below, enter the size of the volume you wish to increase it into.
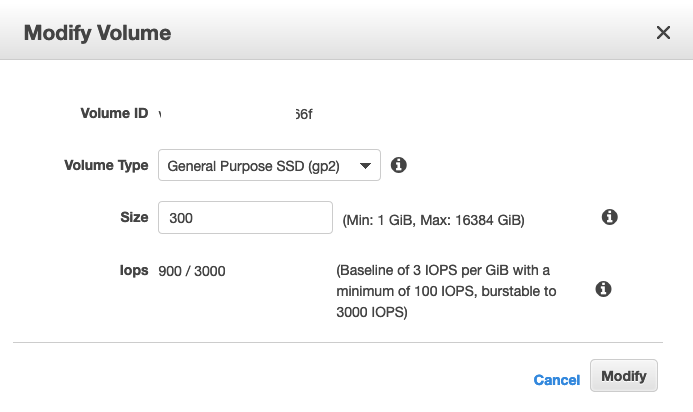
EC2-Modfy-Volume
Once successful you will see the message like below.
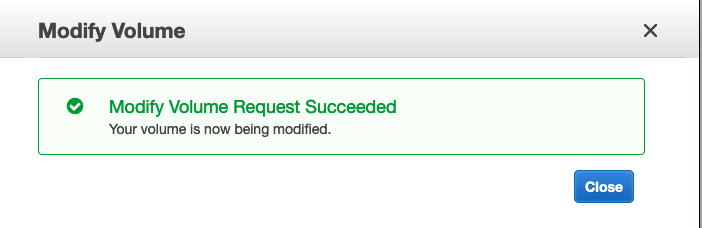
EC2-Modify-Volume-Success
At this point you might be thinking you have completed and it will automagically increase your size. Unfortunately that is not the case, and you will be required to restart your instance and Remote desktop into it to increase it inside of windows also.
We will need to restart the instance and once restarted let us check the disk size.
As it states it is still 300G, we have changed it to 400G but nothing has changed. What the hell???
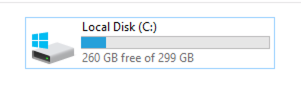
EC2-Windows-Disksize
We will need to launch disk manager (diskmgmt.msc) and we will see that there is 100G that is not allocated yet.
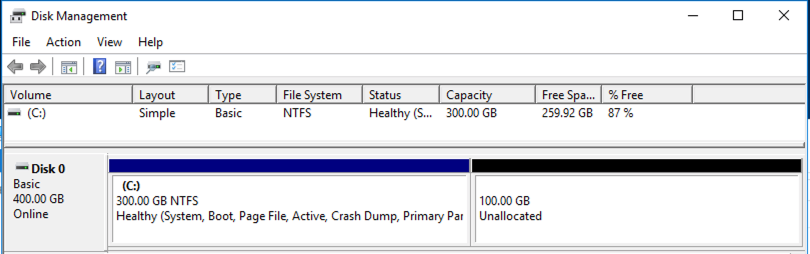
EC2-Unallocated-Space
We will need to right click on our existing volume and choose extend volume like below.
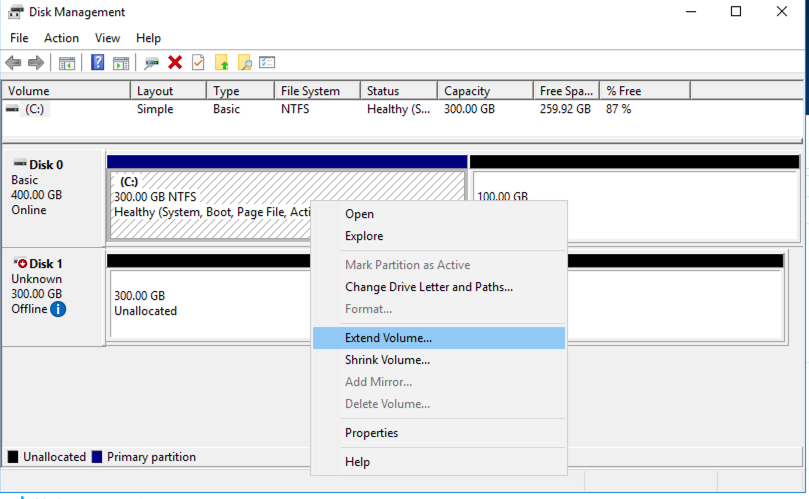
EC2-Extend-Volume
It will bring up a wizard where you click next, next and it will increase your volume like below.
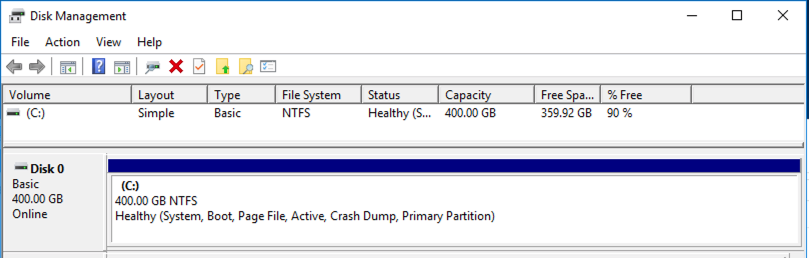
EC2-Final-Result-Extended-Volume
Summary
I hope this helped in showing how to increase the volume size for your windows server running on EC2.